Is this the first time you are about to create a REST API? Then you have arrived at t
- Package fmt- It denotes the Format package. It allows go developers to format inputs, values, basic strings, and outputs. It can also be used from the terminal to print and write. If you are familiar with the C programming language, then you will find it similar to C’s printf and scanf.
- Package log- The standard library of Golang has a built-in log package that offers primitive logging features. The package helps in defining the type Logger with different methods for output formatting.
- Package net/http- If you wish to build HTTP servers in Golang, then you have to use the net/http package. It provides both HTTP server and client implementations. The HTTP request comprises Get, Post, Head and PostForm.
- Package encoding/json- JSON is encoded and decoded using the package json in accordance with RFC 7159. The Marshal and Unmarshal functions' documentation describes the mapping between Golang and JSON values.
Next, we will be creating a struct called ‘Movie’. And we will insert three different fields:
- Title string
- Timelimit int
- Cast string
You will also observe tags like ‘json:”title”’. Well, these struct tags denote what the name of the fields should be when the contents of the struct are serialized into JSON. And you have to use small letters after ‘json’, as it is case sensitive to both data and field names.
- Next we create a slice named ‘Movies’.
- Following this, we create four functions named Intro, returnAllMovies, handleRequests, and main.
- The Intro function will be responsible for handling all the requests to the root URL.
- The HandleRequests function will be taking the help of a defined function to match the URL path.
- The main function will start the API.
- The function returnAllMovies will handle the task of returning the Movies variable, where we will be encoding using the JSON format.
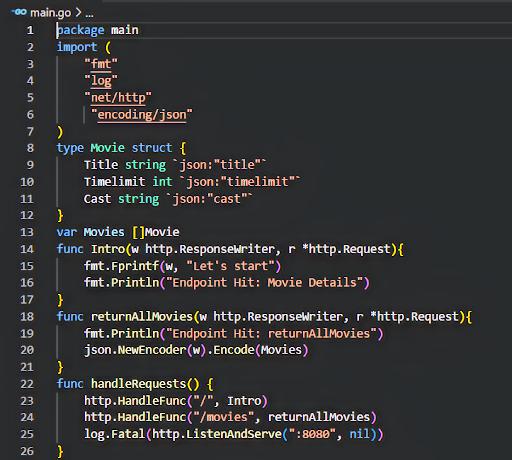
- The argument (w http.ResponseWriter, r *http.Request) in the function Intro helps in reading and writing. And you can use them as you have imported the package net/http, that provides server implementations and HTTP clients.
Then we print out
fmt.Fprintf
(w, “Let’s Start”)
fmt.Println
(“Endpoint Hit: Movie Details”)
- In the function returnAllMovies, we insert the argument (w http.ResponseWriter, r *http.Request). And we focus on marshaling of JSON with the statement json.NewEncoder (w).Encode (Movies). It encodes the Movies slice into the JSON string.
- Then we describe the handleRequests and the most noteworthy thing to remember here is when we create the port with ListenandServe. The latter pays heed to the TCP network address, and it then calls upon Serve in order to handle the incoming connection requests.
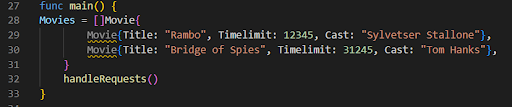
- In the function main, we provide values for the struct ‘Movie’ and we call the function handleRequests.
Executing the Program
- Now, it’s time to run the program. For this, we go to the terminal and type go run main.go.
- Next, we open our browser and type http://localhost:8080
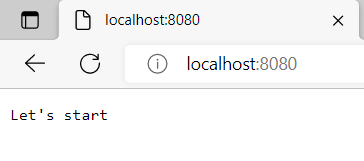
- When we do so we get a ‘Let’s start’ message. This refers to the fact that we have successfully created the base, from which we can develop the REST API.
- Now, we will try to see whether we were able to create the REST endpoint. This means that if we provide the HTTP Get Request, then it all should return the details of the struct Movie that we created. And which we defined in the function ‘main’ by assigning different values.
- In the browser, when we type the address localhost:8080/movies, we get to see the output

[{“title”: “Rambo”, “timelimit”:1245, “cast”:”Sylvester Stallone”}, {“title”:”Bridge of Spies”, “timelimit”: 31245, “cast”:”Tom Hanks”}]
So, we have been able to create the API endpoint successfully. Now, this is a basic representation of creating a REST API. Furthermore, you will get to learn about creating REST API with package gorilla/mux instead of the router net/http. Following this, you will learn to work with the Gin framework, connect MongoDB database and much more.
So, take your time in understanding the concept and process. And you will surely be able to create RESTful APIs without any hassle.